Document capture
About this guide
This guide is designed to assist you in the integration with the Android SDK in a fast and easy way. On this page, you find some key concepts, implementation examples, as well as how to interact with the Biometric Engine REST APIs.
Please note that this guide focuses on the image capture process. For more information about the REST APIs of Unico Check, please refer to REST API Reference guide.
Following this guide, you are able to:
- Learn how to open user's camera and capture an image;
- Learn how to link the parameters returned by the SDK with the REST APIs;
- Learn how to deal with the data returned by the REST API;
Before you begin
The step-by-step instructions on Getting started guide to set up your account, get your API key and install the SDK must be completed. It is also recommended that you check the available features of this SDK on the Overview page.
Available resources
This SDK offers a component that allows you to capture optimized images in your app, displaying to your users a silhouette that gets automatically adjusted to the size of their screens. You can capture the following documents with the SDK:
- Sem silhueta: Captura documento genérico;
- RG: Captura do RG (separado em frente e verso);
- CNH: Captura da CNH aberta;
- CNH frente: Captura da frente da CNH;
- CNH verso: Captura do verso da CNH;
- CPF: Captura do documento de CPF;
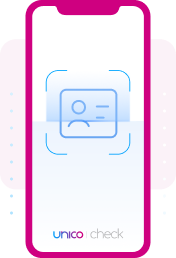
Implementation
Follow the steps below to have the full potential of the SDK embedded in your app.
Initialize the SDK
First, import the SDK and implement the interface
AcessoBioManagerDelegate
inside your ViewController.- Objective-C
- Swift
.m:
#import "ViewController.h"
#import <AcessoBio/AcessoBio.h>
@interface ViewController ()
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
unicoCheck = [[AcessoBioManager alloc]initWithViewController:self];
}
- (void)onErrorAcessoBioManager:(ErrorBio *)error {
code
}
- (void)onSystemChangedTypeCameraTimeoutFaceInference {
code
}
- (void)onSystemClosedCameraTimeoutSession {
code
}
- (void)onUserClosedCameraManually {
code
}import UIKit
import AcessoBio
class ViewController: UIViewController, AcessoBioManagerDelegate {
var unicoCheck: AcessoBioManager!
override func viewDidLoad() {
super.viewDidLoad()
unicoCheck = AcessoBioManager(viewController: self)
}
func onErrorAcessoBioManager(_ error: ErrorBio!) {
// your code
}
func onUserClosedCameraManually() {
// your code
}
func onSystemClosedCameraTimeoutSession() {
// your code
}
func onSystemChangedTypeCameraTimeoutFaceInference() {
// your code
}
}This implementation can be done with just some lines of code. Override the callback functions with your business rules. Each one of the callback functions is invoked as detailed below:
onErrorAcessoBioManager(_ error: ErrorBio!)
MethodThis callback function is invoked whenever an implementation error happens. For example, when informing an incorrect or inexistent capture mode while configuring the camera.
Once invoked, this callback function receives an object of type
ErrorBio
containing the error details. Learn more about the typeErrorBio
in the iOS SDK references document.onUserClosedCameraManually()
MethodThis callback function is invoked whenever an user manually closes the camera. For example, when clicking on the "Back" button.
onSystemClosedCameraTimeoutSession()
MethodThis callback function is invoked whenever the session timeout is reached (Without capturing any image).
Session TimeoutThe session timeout can be set with the builder using the
setTimeoutSession
method. The session timeout must be configured in seconds.Implement delegates to camera events
Through the implementation of delegates, you can configure what happens in your App in both error or success cases when capturing an image, using the methods
onSuccessDocument
oronErrorDocument
, respectively.To configure the delegates, you have to implement the interfaces
DocumentCameraDelegate
andAcessoBioDocumentDelegate
:- Objective-C
- Swift
.h:
#import <UIKit/UIKit.h>
#import <AcessoBio/AcessoBio.h>
#import "SelfieCameraDelegate.h"
@interface ViewController : UIViewController < AcessoBioManagerDelegate,
DocumentCameraDelegate,
AcessoBioDocumentDelegate> {
AcessoBioManager *unicoCheck;
// Your code from previous and next steps here ;)
}import UIKit
import AcessoBio
class ViewController: UIViewController,
AcessoBioManagerDelegate,
DocumentCameraDelegate,
AcessoBioDocumentDelegate {
//Your code from previous and next steps here ;)
}Method
onSuccessDocument
This method is invoked whenever an image is successfully capture. Once invoked, this function receives an object of type
ResultCamera
that is used latter to call the Unico Check REST APIs.- Objective-C
- Swift
- (void)onSuccessDocument:(DocumentResult *)result {
NSLog(@"%@", result.base64);
}
func onSuccessDocument(_ result: DocumentResult!) {
// your code
}A successful response would include the object
ResultCamera
with the following attributes:base64
: This attribute can be used in the case you want to display a preview of the captured image in your app;encrypted
: This attribute must be sent to the unico check REST APIs as detailed here);
Converting a Base64 to BitmapIf you want to convert a Base64 string into a Bitmap image, the standard way won´t work in iOS. Learn more at this Stack Overflow article.
AlertThe Encrypted attribute is strictly intended for sending the image through the Unico APIs. You should not open and serialize this attribute, as its characteristics may change without notice. Its use must be exclusive in interactions with the APIs to guarantee the data integrity and security. Unico is not responsible for any damages arising from this practice, since the changes may occur unpredictably.
Method
onErrorDocument
This method is invoked whenever an error happens while capturing an image. Once invoked, this callback function receives an object of type
ErrorBio
containing the error details. Learn more about the typeErrorBio
at iOS SDK references document.- Objective-C
- Swift
- (void)onErrorDocument:(ErrorBio *)errorBio {
// Your code
}
func onErrorDocument(_ errorBio: ErrorBio!) {
// Your code
}Object ErrorBioLearn more about the type
ErrorBio
at iOS SDK references document.Customize the capture frame
Optional stepThis step is optional but recommended.
We offer you the possibility of customizing the capture frame in the SDK. To customize it, you just need to use the method corresponding to the property to be customized and apply the change with the
setTheme()
method.Learn more about the method
setTheme()
and the customization possibilities at iOS SDK reference documentation.Prepare and open the camera
First, we must prepare the camera using the method
prepareDocumentCamera
that receives as parameter the implementation of the classDocumentCameraDelegate
together with the SDK credentials, configured in this step.- Objective-C
- Swift
.h:
#import <UIKit/UIKit.h>
#import <AcessoBio/AcessoBio.h>
#import "SelfieCameraDelegate.h"
@interface ViewController : UIViewController < AcessoBioManagerDelegate,
DocumentCameraDelegate, AcessoBioDocumentDelegate> {
AcessoBioManager *unicoCheck;
}
.m:
- (IBAction)openCamera:(UIButton *)sender {
// with AcessoBioConfigDataSource implementation
[[unicoCheck build] prepareDocumentCamera:self config: [YourUnicoConfigClass new]];
// or
// with JSON config
[[unicoCheck build] prepareDocumentCamera:self jsonConfigName: @""];
}import UIKit
import AcessoBio
class ViewController: UIViewController, AcessoBioManagerDelegate,
DocumentCameraDelegate, AcessoBioDocumentDelegate {
@IBAction func openCamera(_ sender: Any) {
// with AcessoBioConfigDataSource implementation
unicoCheck.build().prepareDocumentCamera(self, config: YourUnicoConfigClass())
// or
// with JSON config
unicoCheck.build().prepareDocumentCamera(self, jsonConfigName:
"json-credenciais.json")
}
}Once the camera is ready, the method
onCameraReadyDocument
is triggered receiving as parameter an object of typeAcessoBioCameraOpenerDelegate
.You must override this method and open the camera using the method
openDocument()
that receives as parameter:Type of document to be capture:
DocumentEnums.none
: Any kind of document, without any silhouette ;DocumentEnums.RG
: Brazilian regional ID Document (RG) - First front, then back;DocumentEnums.rgFrente
: Brazilian regional ID Document (RG) - Front;DocumentEnums.rgVerso
: Brazilian regional ID Document (RG) - Back;DocumentEnums.CNH
: Brazilian Driver's licence - Opened document, front and back;DocumentEnums.cnhFrente
: Brazilian Driver's licence - Front;DocumentEnums.cnhVerso
: Brazilian Driver's licence - Back;DocumentEnums.CPF
: Brazilian national ID Document (CPF);
The delegates implemented above (Described as Self);
- Objective-C
- Swift
- (void)onCameraReadyDocument:(id)cameraOpener {
[cameraOpener openDocument:DocumentCNH delegate:self];
}
- (void)onCameraFailedDocument:(NSString *)message {
code
}func onCameraReadyDocument(_ cameraOpener: AcessoBioCameraOpenerDelegate!) {
cameraOpener.openDocument(
DocumentEnums.CNH,
delegate: self
)
}
func onCameraFailedDocument(_ message: String!) {
code
}In the case of an error, the event
onCameraFailedDocument
is triggered. You must override this method, handling the exception, with your business rules.A successful response would trigger the event
onSuccessDocument
, as explained at this step.Call the REST APIs
Capturing the images is just the first part of the journey. After capturing the image, it is necessary to send the generated Encrypted to the APIs, selecting one of the available flows detailed in Flows.
AttentionFor security reasons, the interval between generating the Encrypted and sending it via one of the available flows must be a maximum of 10 minutes. Submissions made beyond this period will be automatically rejected by the API.
Getting help
Are you missing something or still need help? Please, please get in touch with the support team at help center.
Next steps
- Learn how to capture Selfies with the SDK.
- Learn more about the SDK in the Reference documentation.
- Check the REST APIs documentation.