Document capture
About this guide
This guide is designed to assist you in the integration with the Android SDK in a fast and easy way. On this page, you find some key concepts, implementation examples, as well as how to interact with the Biometric Engine REST APIs.
Please note that this guide focuses on the image capture process. For more information about the REST APIs of Unico Check, please refer to REST API Reference guide.
Following this guide, you are able to:
- Learn how to open user's camera and capture an image;
- Learn how to link the parameters returned by the SDK with the REST APIs;
- Learn how to deal with the data returned by the REST API;
Before you begin
The step-by-step instructions on Getting started guide to set up your account, get your API key and install the SDK must be completed. It is also recommended that you check the available features of this SDK on the Overview page.
Available resources
This SDK offers a component that allows you to capture optimized images in your app, displaying to your users a silhouette that gets automatically adjusted to the size of their screens. You can capture the following documents with the SDK:
- CPF: Brazilian national ID Document (CPF);
- CNH: Brazilian Driver's licence - Opened document, front and back;
- CNH Front: Brazilian Driver's licence - Front;
- CNH Back: Brazilian Driver's licence - Back;
- RG Front: Brazilian regional ID Document (RG) - Front;
- RG Back: Brazilian regional ID Document (RG) - Back;
- Other: Any kind of document.
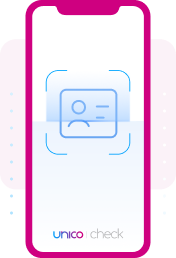
We offer you the possibility of customizing the capture frame in the SDK. To customize it, you just need to use the method corresponding to the property to be customized and apply the change with the setTheme()
method.
Learn more about the setTheme()
method and the customization possibilities at Android´s SDK reference documentation.
Implementation
Follow the steps below to have the full potential of the SDK embedded in your app in just some few minutes:
Initialize the SDK
First, you have to instantiate the builder, through the interface
UnicoCheckBuilder
.- Dart
class _MyHomePageState extends State<MyHomePage> implements UnicoListener {
late UnicoCheckBuilder _unicoCheck;
/// Unico callbacks
@override
void onErrorUnico(UnicoError error) {}
@override
void onUserClosedCameraManually() {}
@override
void onSystemChangedTypeCameraTimeoutFaceInference() {}
@override
void onSystemClosedCameraTimeoutSession() {}
/// Document callbacks
@override
void onSuccessDocument(ResultCamera resultCamera) { }
@override
void onErrorDocument(UnicoError error) { }
}This implementation can be done with just some lines of code. Override the callback functions with your business rules. Each one of the callback functions is invoked as detailed below:
onErrorUnico(UnicoError error)
This callback function is invoked whenever an implementation error happens. For example, when informing an incorrect or inexistent capture mode while configuring the camera.
Once invoked, this callback function receives an object of type
ErrorBio
containing the error details. Learn more about the typeErrorBio
in the Flutter SDK references document.onUserClosedCameraManually()
This callback function is invoked whenever an user manually closes the camera. For example, when clicking on the "Back" button.
onSystemClosedCameraTimeoutSession()
This callback function is invoked whenever the session timeout is reached (Without capturing any image).
Session TimeoutThe session timeout can be set with the builder using the
setTimeoutSession
method. The session timeout must be configured in seconds.Be carefulAll the above callback functions must be declared in your project (Even without any business rules). Otherwise, you won't be able to compile your project.
Open the camera
Finally, let´s open the camera! To make it easier, this last piece is splitted in some steps.
Implementing the listeners
Through the implementation of listeners, you can configure what happens in your App in both error or success cases when capturing an image, using the methods
onSuccessDocument
oronErrorDocument
, respectively.onSuccessDocument
Methodpublic void onSuccessDocument(ResultCamera resultCamera) { }
This method is invoked whenever an image is successfully capture. Once invoked, this function receives an object of type
ResultCamera
that is used latter to call the Unico Check Rest APIs.onErrorDocument
Methodpublic void onErrorDocument(UnicoError error) { }
This method is invoked whenever an error happens while capturing an image. Once invoked, this callback function receives an object of type
ErrorBio
containing the error details. Learn more about the typeUnicoError
at Flutter SDK references document.Listeners implementationThe implementation of these listeners must be done inside an instance of the class
UnicoDocument
.Customize the capture frame
Optional stepThis step is optional but recommended.
We offer you the possibility of customizing the capture frame in the SDK. To customize it, you just need to use the method corresponding to the property to be customized and apply the change with the
setTheme()
method.Learn more about the method
setTheme()
and the customization possibilities at Flutter SDK reference documentation.Abrir a câmera
Finally, we must open the camera with the settings done until this step. To that, we must call the
openCameraDocument()
method.Then, we must open the camera using the
openCameraDocument()
method, that was generated by the object from the classUnicoCheck
. This method must receive as parameter:- JSON file with your credentials, generated at this step.
- Type of document to be capture:
DocumentCameraType.CPF
: Brazilian national ID Document (CPF);DocumentCameraType.CNH
: Brazilian Driver's licence - Opened document, front and back;DocumentCameraType.CNH_FRENTE
: Brazilian Driver's licence - Front;DocumentCameraType.CNH_VERSO
: Brazilian Driver's licence - Back;DocumentCameraType.RG_FRENTE
: Brazilian regional ID Document (RG) - Front;DocumentCameraType.RG_VERSO
: Brazilian regional ID Document (RG) - Back;DocumentCameraTypes.NONE("description")
: Any kind of document, without silhouette. In this case you must include the name of the document as a description to be displayed in the frame;
- The listeners configured above;
Example capturing a CNH:
- Dart
_unicoCheck.build().openCameraDocument(
jsonFileName: androidJsonFileName,
documentType: DocumentType.CNH,
listener: this);A successful response would include the object
ResultCamera
with the following attributes:base64
: This attribute can be used in the case you want to display a preview of the captured image in your app;encrypted
: This attribute must be sent to the unico check REST APIs as detailed here;
Call the REST APIs
The image capture is just the first step of the journey. Now, you have to send the obtained
JWT
to the Rest APIs using one of the available flows, detailed in this page.
Getting help
Are you missing something or still need help? Please, please get in touch with the support team at help center.
Next steps
- Learn how to capture Selfies with the SDK.
- Learn more about the SDK in the Reference documentation.
- Check the REST APIs documentation.