WKWebView
For iOS usage scenario, using the WKWebView is one of the recommended ways.
After creating the transaction and getting the transaction link, the following implementation is recommended:
- In your common flow (which IDPay is inserted) you will open the WKWebView with the link generated through the API;
- Customize this opening in any way that is ideal for your application;
- Monitor if the URL has changed (to redirectUrl) and then close the WKWebView;
The following code shows how to open a WKWebView:
import UIKit
import WebKit
import SafariServices
var webView: WKWebView!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
self.view.backgroundColor = .white
}
@IBAction func open(_ sender: Any) {
createWKWebViewFull()
}
func createWKWebViewFull() {
webView = WKWebView(frame: self.view.bounds, configuration: getWKWebViewConfiguration())
webView.navigationDelegate = self
view.addSubview(webView)
self.loadUrl()
}
func loadUrl() {
if let url = URL(string:"<URL_TO_LOAD>") {
webView.load(URLRequest(url: url))
webView.allowsBackForwardNavigationGestures = true
}
}
private func getWKWebViewConfiguration() -> WKWebViewConfiguration {
let config = WKWebViewConfiguration()
config.allowsInlineMediaPlayback = true
config.mediaTypesRequiringUserActionForPlayback = []
return config
}
The following code shows how to control when to close the WKWebView:
func webView(_ webView: WKWebView, didCommit navigation: WKNavigation!) {
if let currentURL = webView.url {
print("URL changed to: \(currentURL.absoluteString)")
if currentURL.absoluteString == "<URL_TO_OBSERVER>" {
closeWebView()
}
}
}
@objc func closeWebView() {
DispatchQueue.main.asyncAfter(deadline: .now() + 0.1) {
self.webView.removeFromSuperview()
}
}
Follows an example of how it should look in the APP:
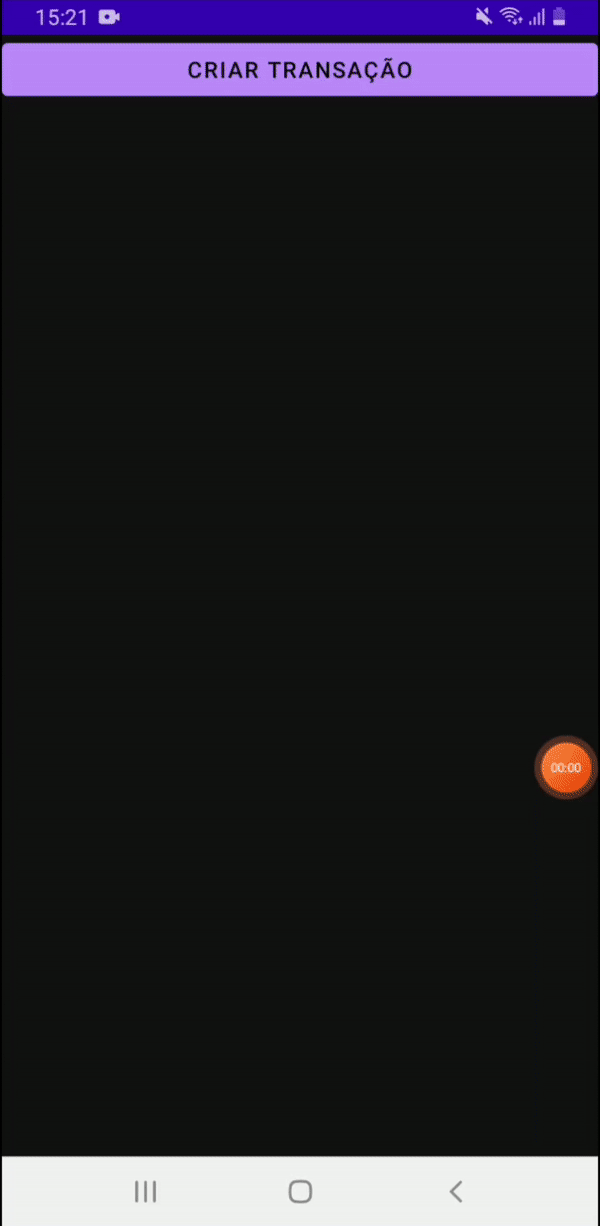
To know more about the WKWebView, the following articles and documentation are recommended:
- Access the official documentation at WKWebView;
IMPORTANT
The following permissions are required to function correctly:
- Camera;
- Geolocation;
Any concerns?
Missing something or still need help? If you are already a customer or partner, you can contact us through the Help Center.