Android
For Android usage scenario, using the Webview is recommended.
After creating the transaction and getting the transaction link, the following implementation is recommended:
- In your common flow (which IDPay is inserted) you will open the Webview with the link generated through the API;
- Customize this opening in any way that is ideal for your application;
- Monitor if the URL has changed (to redirectUrl) and then close the Webview;
The following code shows how to open a Webview:
import android.webkit.PermissionRequest
import android.webkit.WebChromeClient
import android.webkit.WebView
import android.webkit.WebViewClient
@SuppressLint("SetJavaScriptEnabled")
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_camera)
findViewById<WebView>(R.id.webView).apply {
settings.javaScriptEnabled = true
settings.domStorageEnabled = true
settings.mediaPlaybackRequiresUserGesture = false
clearCache(true)
webViewClient = myWebViewClient
webChromeClient = object : WebChromeClient() {
override fun onPermissionRequest(request: PermissionRequest?) {
request?.grant(request.resources)
}
}
loadUrl("<URL_TO_LOAD>")
}
}
The following code shows how to control when to close the Webview:
private val myWebViewClient = object : WebViewClient() {
override fun shouldOverrideUrlLoading(
view: WebView?,
request: WebResourceRequest?
): Boolean {
if (request?.url.toString() == "<URL_TO_OBSERVER>") {
// close here your webview
}
return super.shouldOverrideUrlLoading(view, request)
}
}
The following permissions must be enabled in the Android Manifest:
<uses-feature android:name="android.hardware.camera" android:required="false"/>
<uses-permission android:name="android.permission.CAMERA"/>
Follows an example of how it should look in the APP:
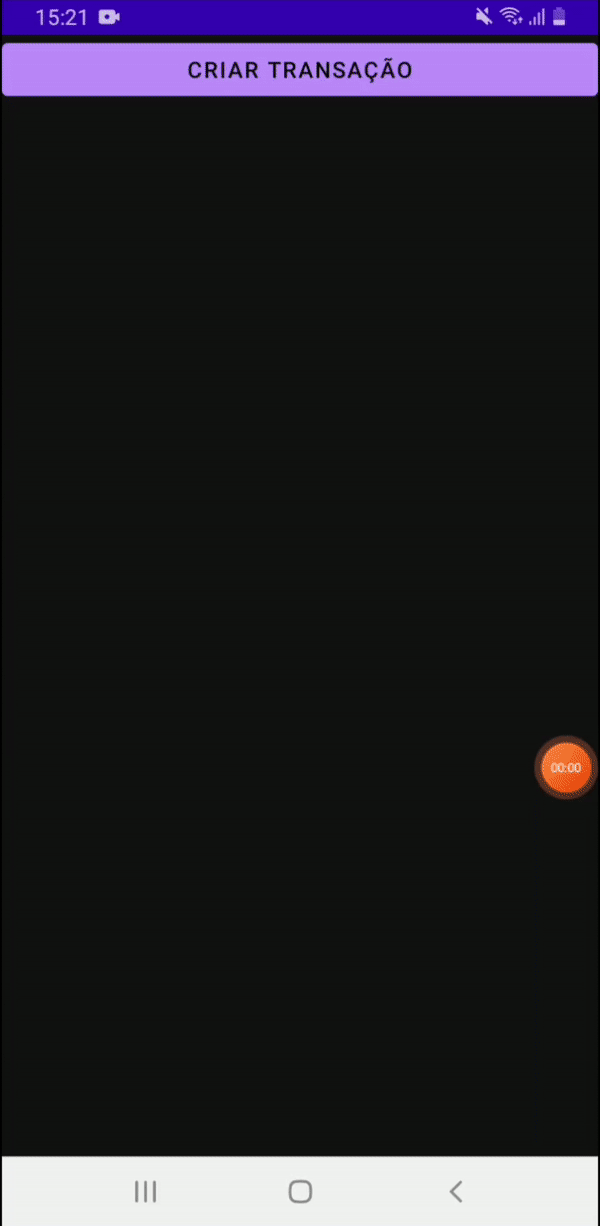
IMPORTANT
The following permissions are required to function correctly:
- Cmera;
- Geolocation;
Any concerns?
Missing something or still need help? If you are already a customer or partner, you can contact us through the Help Center.