Preparing to make an authenticated API request
After creating and configuring a service account, your application needs to complete the following steps:
- Create a JSON Web Token (JWT), which includes header, payload and signature;
- Request an access token (AccessToken) from the OAuth2 authentication platform;
- Handle the JSON response returned by the authentication platform.
If the response includes an access token, you can use it to make requests to the APIs of unico products for which the service account has access permission. If the response does not include an access token, the JWT and request to obtain the token may be incorrect or the service account may not have the necessary permissions to access the requested resources.
The generated access token has a default validity of 3600 seconds. This validity may vary depending on the security configuration established for your company. When the access token expires, your application must generate a new JWT, sign it and request a new access token from the authentication platform.
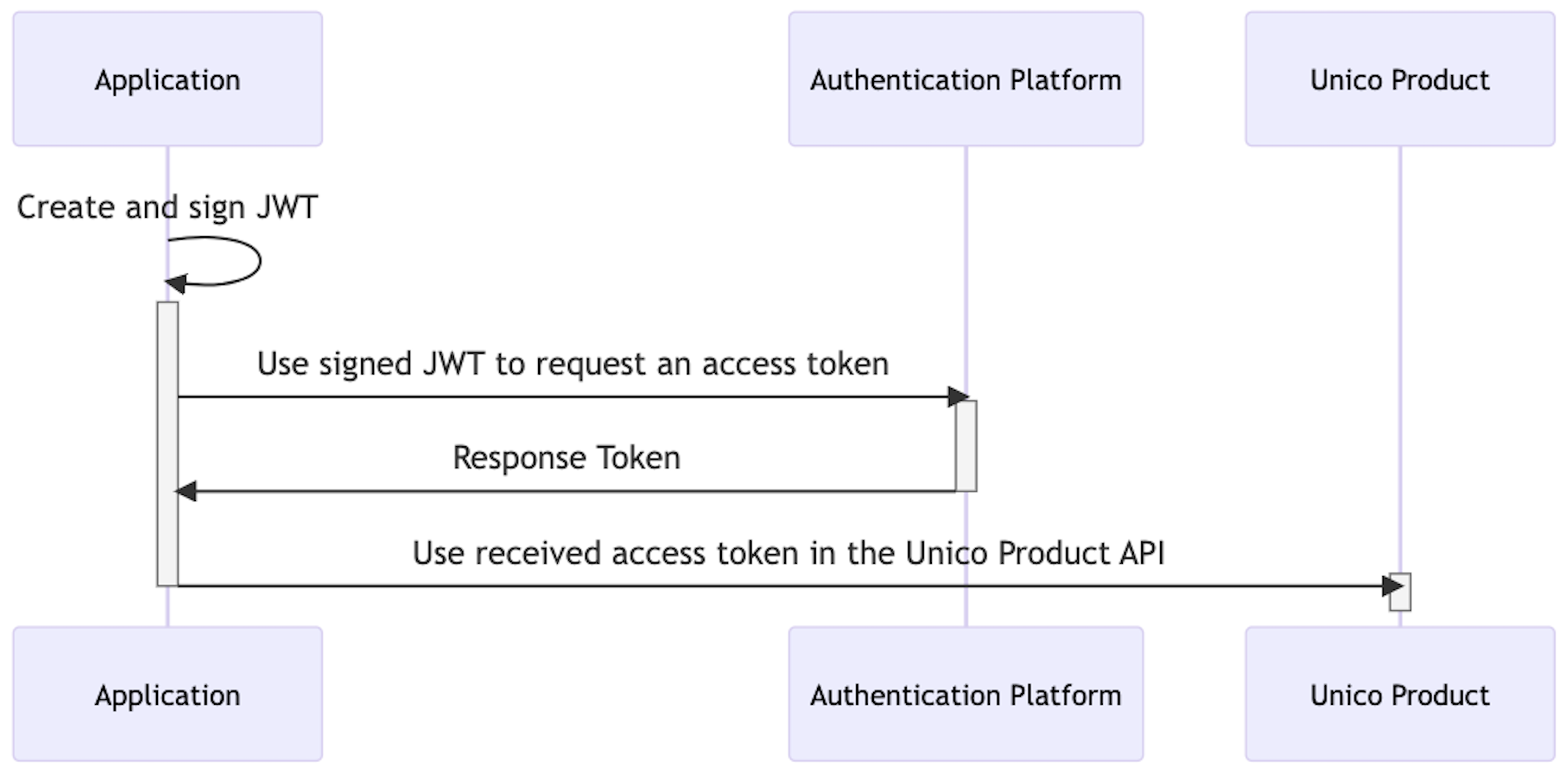
1 - Creating the JWT
A JWT is made up of three parts: a header, a payload, and a signature. The header and the payload are JSON objects. These JSON objects are serialized in UTF-8 and then encoded using Base64url encoding¹. This encoding provides resilience against encoding changes in cases of repeated encoding operations. The header, the payload, and the signature are concatenated with a period .
character.
A JWT is composed as follows:
{Header in Base64url}.{Payload in Base64url}.{Signature in Base64url}
The base text for the signature is composed as follows:
{Header in Base64url}.{Payload in Base64url}
1.1 - Forming the JWT header
The header consists of two fields that indicate the signature algorithm and the token format. The both fields are mandatory and each field has only one value. Service accounts rely on the RSA SHA-256 algorithm and the JWT token format. As a result, the JSON representation of the header is as follows:
{"alg":"RS256","typ":"JWT"}
The representation in Base64url is as follows:
eyJhbGciOiJSUzI1NiIsInR5cCI6IkpXVCJ9
1.2 - Forming the JWT payload
The payload contains the JWT information, including the permissions being requested (scopes), the account requesting access, the sender, when the token was sent, and the token lifetime. Most fields are mandatory. The payload is a JSON object and it is used in the signature composition.
1.3 - Mandatory fields
The mandatory fields in JWT are shown in the following table. They can appear in any order within the payload.
Name | Description |
---|---|
iss | The service account identifier in the company. |
scope | A list of permissions required by the application. The list is delimited by spaces or the plus sign + . If all account permissions are required, use the asterisk * sign |
aud | Address of the authentication platform that sends access tokens. This value must be https://identityhomolog.acesso.io Attention to the possible errors: - Insertion of a slash at the end of the address: https://identityhomolog.acesso.io/ - Using HTTP protocol instead of HTTPS: http://identityhomolog.acesso.io |
exp | Token expiration time, specified in seconds since 00:00:00 UTC, January 1, 1970. This value is valid for 1 hour after sending the JWT. This value must be numeric. Attention to the possible error: The use of quotes in the delimiting of the value making it a string. For example: “1524161193”. |
iat | The time the JWT was sent, specified in seconds since 00:00:00 UTC, January 1, 1970. This value must be numeric. Attention to the following error: The use of quotes in the delimiting of the value making it a string. For example: “1524161193”. |
Click here to understand the conversion of the jwt send (iat) and expiry (exp) fields, and see examples of using field values. The value of the “iat” field must be the current time in the required format and the “exp” must respect the following calculation:
exp = iat + 3600
The representation of the required JSON fields in the JWT payload is as follows:
{
"iss": "service_account_name@tenant_id.iam.acesso.io",
"aud": "https://identityhomolog.acesso.io",
"scope": "*",
"exp": 1626296976, // This is just an example. Use the current generated value here.
"iat": 1626293376 // This is just an example. Use the current generated value here
}
1.4 - Calculating the signature
The JSON Web Signature (JWS)² specification is the mechanics that guide the signature calculation for a JWT. The input content for the signature calculation is the byte array of the following content:
{Header in Base64url}.{Payload in Base64url}
The same algorithm used in the JWT header is also used for the signature calculation. The signature algorithm supported by the OAuth2 authentication platform is the RSA using SHA-256. It is expressed as RS256 in the alg field of the JWT header.
Sign the UTF-8 representation of the input content with the private key that was created and associated with the service account (.key.pem file generated by the request received by email). For signing, use SHA256withRSA (also known as RSASSA-PKCS1-V1_5-SIGN with the SHA-256 hash). The output content is a byte array.
The signature is Base64url encoded. The header, payload, and signature are concatenated with the period .
character. The result is the JWT as follows:
{Header in Base64url}.{Payload in Base64url}.{Signature in Base64url}
The following example denotes the JWT token before Base64url encoding:
{"alg":"RS256","typ":"JWT"}.
{
"iss": "service_account_name@tenant_id.iam.acesso.io",
"aud": "https://identityhomolog.acesso.io",
"scope": "*",
"exp": 1626296976, // This is just an example. Use the current generated value here.
"iat": 1626293376 //This is just an example. Use the current generated value here.
}.
[byte array da assinatura]
The following example denotes the JWT signed:
eyJhbGciOiJSUzI1NiIsInR5cCI6IkpXVCJ9.eyJpc3MiOiJzZXJ2aWNlX2FjY291bnRf
bmFtZUB0ZW5hbnRfaWQuaWFtLmFjZXNzby5pbyIsImF1ZCI6Imh0dHBzOi8vaWRlbnRpdHlob21vbG9nLmFjZXNzby5pbyIsInNjb
3BlIjoiKiIsImV4cCI6MTYyNjI5Njk3NiwiaWF0IjoxNjI2MjkzMzc2fQ.JsymP3NZdgCSqeNlgsOM2
-AQ7M450NxFnZnnaKSu4Q8g12QGEIvvM4EhCokUHfwk5s7pOpm2UD_Ng3Hb5g_wgrjfiVSLWH5Q2wYg1AvfLqo
YSoJWaMHm9KL0kpv32XdDD8TZVR-MVd2VBHmCMVbV6gvk8buUoK1HZDN7g84PaY3bfgcB3RKU-
H55lR8yyJjZxToIv17-wfla2G99uaMEFNGX0ZSE7ETn5Z8-WypmFrNAK0TM58upzvfVI6_-
gY4cj4iQvmRbuvxsAaGiHA2xd0RVm2Mrx-gQtdPqtbZPuQcH7k64Z_EOQBgiGTgVjucyHD6zBijr_P-
2mhIxuecNSw
It is also possible to use existing libraries to create the JWT. For reference, see the list of libraries on the jwt.io website.
2 - Requesting an access token
With the JWT signed, the application can use it to request an Access Token. This is an HTTPS POST request and the body must be URL encoded. Following the URL:
https://identityhomolog.acesso.io/oauth2/token
The following parameters are mandatory in the POST HTTPS request
Name | Description |
---|---|
grant_type | Use the following text, URL-encoded if necessary: urn:ietf:params:oauth:grant-type:jwt-bearer |
assertion | The JWT, including the signature. |
POST /oauth2/token HTTP/1.1
Host: identityhomolog.acesso.io
Content-Type: application/x-www-form-urlencoded
grant_type=urn%3Aietf%3Aparams%3Aoauth%3Agrant-type%3Ajwt-
bearer&assertion=eyJhbGciOiJSUzI1NiIsInR5cCI6IkpXVCJ9.eyJpc3MiOiJzZXJ2aWNlX2FjY
291bnRfbmFtZUB0ZW5hbnRfaWQuaWFtLmFjZXNzby5pbyIsInN1YiI6InVzZXJfaWRlbnRpZmllciIs
ImF1ZCI6Imh0dHBzOi8vaWRlbnRpdHlob21vbG9nLmFjZXNzby5pbyIsInNjb3BlIjoiKiIsImV4cCI
6MTMyODU1NDM4NSwiaWF0IjoxMzI4NTUwNzg1fQ.TjH-mTtwP6tBB93O1sDPaAA6yUF7N2-HZDlpIPz
bf_dxO8A6KZuRWG8ZnICrxX56qj0HREiMlYy27XJgxowrUa0JHvbqc8HJkT7-6Mh7J67UnubZKG1-hi
6jDtkC9BIXBcOhtkNUfZvZetXjLzsRsSDkqxdMtzYZwkRlocvaxL5QXiQhweeEwK_Ux81Adh3z0EIhT
yl7CKJLJ69PuHS7s9IdrjUl79owipp4LF1FvtMhoe7YIL69ohPgFqSv_-Y9qpPdW6be3OEAyKlOM08S
ZBbHBwW4XMvw3uZjTY1sgJ4cBoxrftDpjYOw34oPOKxirqc5-90uOCYe1O1hRtG45w
3 - Handling the response from the authentication platform
The response of the authentication platform returns a JSON object containing an access token if the JWT and access token request are formed correctly and the service account has the necessary permissions. The following is an example response from the platform:
{
"access_token": "<access_token>",
"token_type": "Bearer",
"expires_in": "3600"
}
The value returned in the “acess_token” field of the JSON object is a JWT token that must be used in the APIs of unico Products. If the request returns an error, check the type of error in the error table here.
4 - Access Token Validity Time
The validity of the access token is variable. The duration time is specified in the “expires_in” field, which is returned with the access token. The same access token must be used while the lifetime is valid for all product API calls.
Do not request a new access token until the current token expires. As a suggestion, request 600 seconds (10 minutes) in advance. How to do the calculation:
decoded token:
new Date(token.exp - 600)
Where token.exp is the token expiration timestamp.
By default, the token sent to the company lasts for 1 hour, but it can be changed. The suggestion is to use the expires_in as a base and then subtract the 600 seconds from it to request a new token.
Examples:
Standard scenario:
expires_in: 3600 (1h) - Token generation at 2:42 pm
Request a new token only at 3:32 pm i.e. 2:42 pm + (3600 - 600)
Scenario with changed duration:
expires_in: 7200 (2h) - Token generation at 2:42 pm
Request a new token only at 4:32 pm i.e. 2:42 pm + (7200 - 600)
A new access token can be requested when when the expiration time is 10 minutes.
Do not use a fixed time to get a new token. If the validity of the received token is less than the established time, it will cause failure to use the services.
5 - Authentication errors
The errors returned in the request have the following structure:
{
"error": "server_error",
"error_description": "Falha na autenticação x.x.x"
}
Code | Description |
---|---|
1.0.14 | Check with the person responsible for the project if the application used is active. |
1.1.1 | Missing the "scope" parameter in the jwt token payload. |
1.2.4 | The jwt token is expired. Check the value of the "exp" field in the payload. |
1.2.5 | The jwt token cannot be validated. Check the parameters informed and if the token signature is correct. |
1.2.6 | The private key used in the jwt token signing is not valid. Request new credentials for the account. |
1.2.7 | The jwt token has already been used. Generate a new token for the new request. |
1.2.11 | The account is inactive. |
1.2.14 | The account does not have the required permissions. |
1.2.18 | The account is temporarily blocked. Too many invalid authentication attempts. |
1.2.19 | The account is not authorized to impersonate another user account. Remove the "sub" parameter from the payload. |
1.2.20 1.2.21 | Jwt token decryption failed. Use a new token with "Required Fields" and "Additional Fields" only. Pay attention to the nomenclature, semantics, and type of each field. |
1.2.22 | The jwt token has additional fields that are not allowed. Use a new token with "Required Fields" and "Additional Fields" only. Pay attention to the nomenclature, semantics, and type of each field. |
1.3.1 | The account has source IP restrictions. |
1.3.2 | The account has access date/time restrictions. |
- ¹ According to the BaseN encoding RFC 4648, the Base64url format is similar to the Base64 format. Exceptions: character = must be omitted, and characters + and / must be replaced by - and _, respectively
- ² JSON Web Signature: https://tools.ietf.org/html/rfc7515